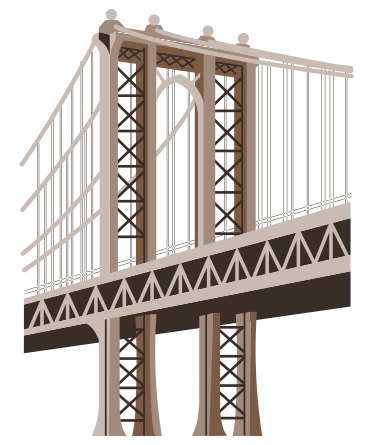
Bridge Widget to świetne narzędzie do komunikacji między różnymi urządzeniami IoT w ramach projektu BLYNK.
Pobiera on wirtualny pin (dowolny, który zadeklarujemy) i zamienia go w kanał do sterowania innym urządzeniem. Oznacza to, że możemy z jednej płytki sterować dowolnymi cyfrowymi, analogowymi lub wirtualnymi pinami drugiej. Trzeba tylko znać jej kod autentyczności. Kody są banalnie proste. Podobno to najrzadziej używany widget. Nie mam pojęcia dlaczego.
Komunikacja master -> slave
KOD dla master:
/*************************************************************
Control another device using Bridge widget!
Bridge is initialized with the token of any (Blynk-enabled) device.
After that, use the familiar functions to control it:
bridge.digitalWrite(8, HIGH)
bridge.digitalWrite("A0", LOW) // <- target needs to support "Named pins"
bridge.analogWrite(3, 123)
bridge.virtualWrite(V1, "hello")
*************************************************************/
#define BLYNK_PRINT Serial
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
#include "config2.h" //auth, ssid, pass
// The first bridge widget on virtual pin 51
WidgetBridge bridge1(V51);
// The second bridge widget on virtual pin 52
WidgetBridge bridge2(V52);
// Timer for blynking
BlynkTimer timer;
static bool value = true;
void blynkAnotherDevice() // Here we will send HIGH or LOW once per second
{
// Send value to another device
if (value) {
bridge1.digitalWrite(2, HIGH); // Digital Pin 2 (LED) włączona na pierwszym urządzeniu
bridge1.virtualWrite(V5, 1); // Sends 1 value to BLYNK_WRITE(V5) handler on receiving side.
bridge2.digitalWrite(13, HIGH); // Digital Pin 13 - stan wysoki na drugim urządzeniu
} else {
bridge1.digitalWrite(2, LOW); // Digital Pin 9 on the second board will be set LOW
bridge1.virtualWrite(V5, 0); // Sends 0 value to BLYNK_WRITE(V5) handler on receiving side.
bridge2.digitalWrite(13, LOW); // Digital Pin 13 - stan niski na drugim urządzeniu
}
// Toggle value
value = !value;
}
BLYNK_CONNECTED() {
bridge1.setAuthToken("..........."); // Wpisz AuthToken pierwszego urządzenia
bridge2.setAuthToken("..........."); // Wpisz AuthToken drugiego urządzenia
}
void setup()
{
// Debug console
Serial.begin(9600);
Blynk.begin(auth, ssid, pass);
// Call blynkAnotherDevice every second
timer.setInterval(1000L, blynkAnotherDevice);
}
void loop()
{
Blynk.run();
timer.run();
}
KOD dla slave:
#define BLYNK_PRINT Serial
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
#include "config.h" //auth, ssid, pass
// This code will update the virtual port 5
BLYNK_WRITE(V5) {
int pinData = param.asInt();
Serial.print(pinData);
}
void setup(){
Serial.begin(9600);
Blynk.begin(auth, ssid, pass);
}
void loop(){
Blynk.run();
}
Komunikacja w obie strony
Urządzenie A
// Urządzenie A
/*************************************************************
Control another device using Bridge widget!
Bridge is initialized with the token of any (Blynk-enabled) device.
After that, use the familiar functions to control it:
bridge.digitalWrite(8, HIGH)
bridge.digitalWrite("A0", LOW) // <- target needs to support "Named pins"
bridge.analogWrite(3, 123)
bridge.virtualWrite(V1, "hello")
*************************************************************/
#define BLYNK_PRINT Serial
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
#include "config.h" //auth, ssid, pass
// Bridge widget on virtual pin 100
WidgetBridge bridge1(V100);
// Timer for blynking
BlynkTimer timer;
static bool value = true;
void blynkAnotherDevice()
{
// Send value to another device
if (value) {
bridge1.digitalWrite(2, HIGH); // D1 mini zapala LED na pinie 2 gdy dostaje niski poziom
bridge1.virtualWrite(V5, "Wyłączone \n");
} else {
bridge1.digitalWrite(2, LOW); // Digital Pin 9 on the second board will be set LOW
bridge1.virtualWrite(V5, "Włączone \n");
}
// Toggle value
value = !value;
}
BLYNK_CONNECTED() {
bridge1.setAuthToken("A4GDZ4YWnRfmGTQlebAEUV1hghRMlSk-"); // Place the AuthToken of the second hardware here
}
BLYNK_WRITE(V8) {
String pinData = param.asString();
Serial.print(pinData);
}
void setup()
{
// Debug console
Serial.begin(9600);
//Blynk.begin(auth, ssid, pass);
Blynk.begin(auth, ssid, pass, IPAddress IPA); // serwer lokalny
// Call blynkAnotherDevice every 3 second
timer.setInterval(3000L, blynkAnotherDevice);
}
void loop()
{
Blynk.run();
timer.run();
}
Urządzenie B
// Urządzenie B
#define BLYNK_PRINT Serial
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
#include "config.h" //auth, ssid, pass
WidgetBridge bridge1(V100);
BlynkTimer timer;
static bool value = true;
void sendMessage(){
if (value){
bridge1.virtualWrite(V8, "Komunikat ");
}else{
bridge1.virtualWrite(V8, "zwrotny.\n");
}
value=!value;
}
// This code will update the virtual port 5
BLYNK_WRITE(V5) {
String pinData = param.asString();
Serial.print(pinData);
}
BLYNK_CONNECTED() {
bridge1.setAuthToken("ekkoLIeyEgwhbMJ0KW53Hhk4X4q3nJ8R"); // Place the AuthToken of the second hardware here
}
void setup(){
Serial.begin(9600);
//Blynk.begin(auth, ssid, pass, IPAddress(192,168,0,5), 8080);
Blynk.begin(auth, ssid, pass, IPAddress IPA);
timer.setInterval(3000L, sendMessage);
}
void loop(){
Blynk.run();
timer.run();
}